# Database
# Create
1 | create database MarketManagement; |
# Use
1 | use MarketManagement; |
# Table
# Create
-
If the column name includes a keyword or includes a space, enclose it in brackets, such as [Launch Date].
-
A primary key constraint can be located after the column definition or can be defined separately after all the column definitions.
-
Char is fixed length. If the inserted data length is less than the fixed length, it will pad space at the end. Such as the Category is char(6) , if you insert
'MEAT'
which is only 4 characters, the data will be stored as'MEAT '
. -
decimal [ ( p [ , s ] ) ] and numeric [ ( p [ , s ] ) ]
-
p (precision)
The maximum total number of decimal digits to be stored. This number includes both the left and the right sides of the decimal point. The precision must be a value from 1 through the maximum precision of 38. The default precision is 18.
-
s (scale)
The number of decimal digits that are stored to the right of the decimal point. This number is subtracted from p to determine the maximum number of digits to the left of the decimal point. Scale must be a value from 0 through p, and can only be specified if precision is specified. The default scale is
0
, and so0 <= s <= p
. Maximum storage sizes vary, based on the precision.
If
99.9999
is inserted into a column with type Decimal (18,2), the data will be rounded up to100.00
. Conversely, if99.444
, is inserted, the data will be rounded down to99.44
. -
1 | create table Products ( |
# Get Create Table Script
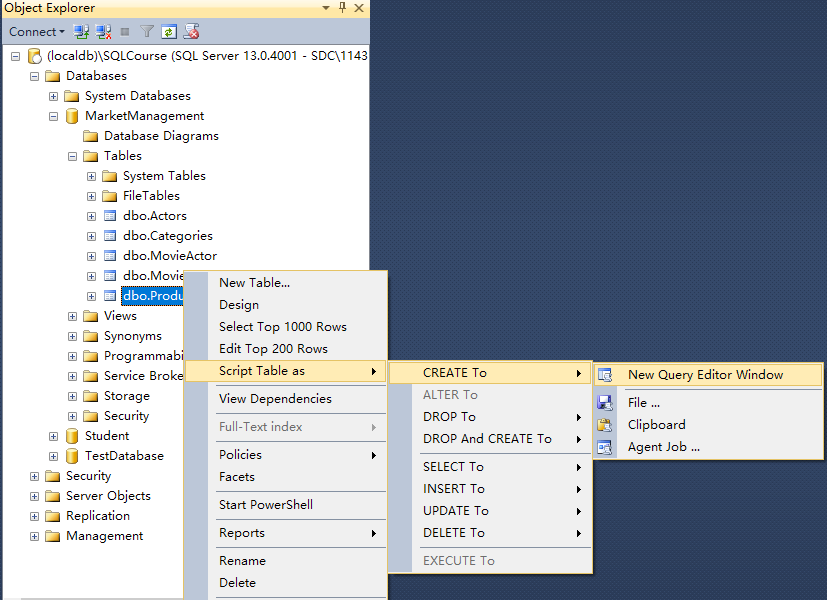
1 | USE [MarketManagement] |
# View Table Details
1 | exec sp_help Products; |
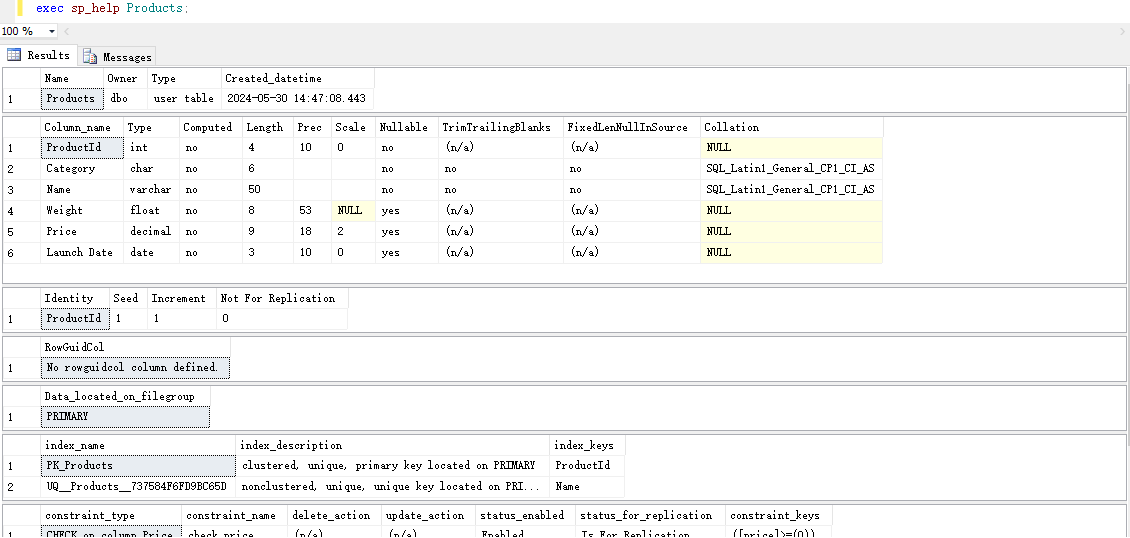
# Insert
1 | insert into Products |
# Select
1 | select * from Products; |
ProductId | Category | Name | Weight | Price | Launch Date |
---|---|---|---|---|---|
1 | MEAT |
Beef | 99.9999 | 100.00 |
2024-05-30 |
2 | MEAT |
Pork | 99.9999 | 99.99 | 2024-05-30 |
# Select * Expand
select Columns
and drag to the destination.
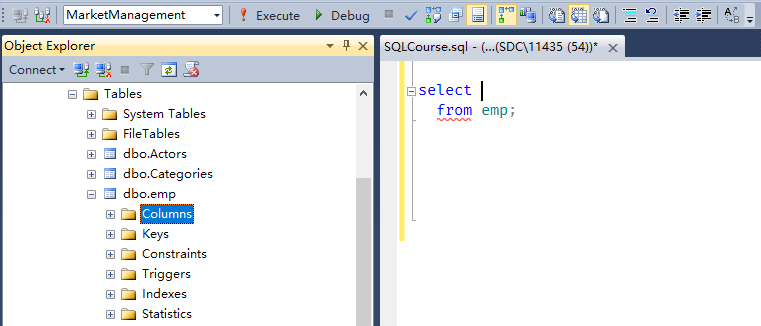
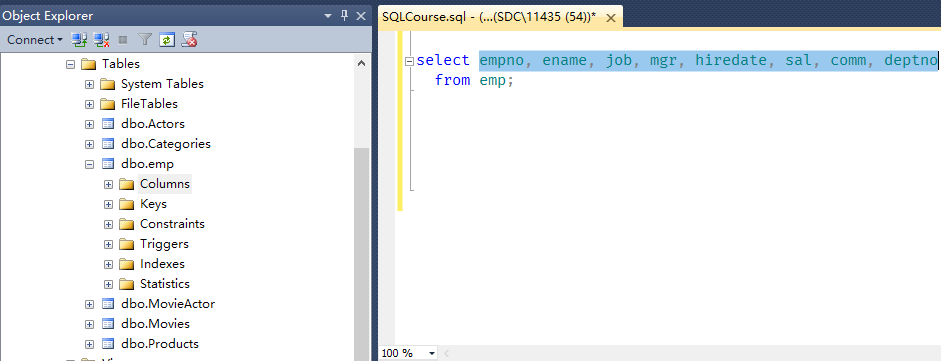
# Top
1 | select top 1 * from Products; |
# Union and Order by
order by execute after union.
1 | select 2 |
1 | select 2 |
# Join
1 | DROP TABLE IF EXISTS LeftTable |
1 | SELECT * FROM LeftTable |
Id | Name |
---|---|
1 | Red |
2 | Green |
3 | Blue |
4 | Yellow |
5 | Purple |
1 | SELECT * FROM RightTable |
Id | ReferenceId | Name |
---|---|---|
1 | 1 | Dog |
2 | 1 | Cat |
3 | 2 | Bird |
4 | 4 | Horse |
5 | 3 | Bear |
6 | 1 | Deer |
7 | 7 | Fish |
# Inner Join
1 | SELECT L.Name, |
Name | Name |
---|---|
Red | Dog |
Red | Cat |
Green | Bird |
Yellow | Horse |
Blue | Bear |
Red | Deer |
# Left Outer Join
1 | SELECT L.Name, |
Name | Name |
---|---|
Red | Dog |
Red | Cat |
Red | Deer |
Green | Bird |
Blue | Bear |
Yellow | Horse |
Purple | NULL |
# Right Outer Join
1 | SELECT L.Name, |
Name | Name |
---|---|
Red | Dog |
Red | Cat |
Red | Deer |
Green | Bird |
Blue | Bear |
Yellow | Horse |
NULL | Fish |
# Full Outer Join
1 | SELECT L.Name, |
Name | Name |
---|---|
Red | Dog |
Red | Cat |
Red | Deer |
Green | Bird |
Blue | Bear |
Yellow | Horse |
Purple | NULL |
NULL | Fish |
# Cross Apply
Cross Apply is similar to Inner Join.
1 | SELECT L.Name, |
Cross Apply with a Table Expression.
1 | SELECT * |
Id | Name | Name |
---|---|---|
1 | Red | Deer |
1 | Red | Cat |
2 | Green | Bird |
3 | Blue | Bear |
4 | Yellow | Horse |
Cross Apply with a Table-valued Functions.
1 | CREATE OR ALTER FUNCTION dbo.GetTopAnimal |
Id | Name | Name |
---|---|---|
1 | Red | Deer |
1 | Red | Cat |
2 | Green | Bird |
3 | Blue | Bear |
4 | Yellow | Horse |
# Outer Apply
Outer Apply is similar to Left Outer Join.
1 | SELECT L.Name, |
# Update
# Multi Table Join Update
1 | update edi_head |
# Alter
1 | exec sp_rename Products,Product; --rename table name |
# Table Relation
# Many to Many
1 | create table Movies |
# Temp Table & Table Variables
1 | create table #edi_temp ( |
1 | declare @emp table ( |
# Constraint
- sys.key_constraints: 这个视图包含了主键约束和唯一键约束的信息。
- sys.check_constraints: 这个视图包含了检查约束的信息。
- sys.default_constraints: 这个视图包含了默认约束的信息。
- sys.foreign_keys: 这个视图包含了外键约束的信息。
1 | -- 查询表的主键约束信息 |
In SQLServer not null
constraint can not find by view and default
is a constraint.
1 | create table edi_test( |
constraint_name | table_name | column_name | default_definition |
---|---|---|---|
DF__edi_test__b__2E1BDC42 |
edi_test | b | (’-’) |
Drop column need drop default
constraint firstly but not null constraint not need.
1 | alter table dbo.edi_test drop column b; --SQL Error [5074] [S1000]: The object 'DF__edi_test__b__2E1BDC42' is dependent on column 'b'. |
In Oracle not null
constraint can not find by view and default
is not a constraint.
1 | create table edi_test( |
OWNER | CONSTRAINT_NAME | CONSTRAINT_TYPE | TABLE_NAME | SEARCH_CONDITION | R_OWNER | R_CONSTRAINT_NAME | DELETE_RULE | STATUS | DEFERRABLE | DEFERRED | VALIDATED | GENERATED | BAD | RELY | LAST_CHANGE | INDEX_OWNER | INDEX_NAME | INVALID | VIEW_RELATED |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
APPS | SYS_C001485655 | C | EDI_TEST | “A” IS NOT NULL | ENABLED | NOT DEFERRABLE | IMMEDIATE | VALIDATED | GENERATED NAME | 2024-03-30 03:52:07 PM |
Drop column not need drop not null
constraint firstly.
1 | alter table edi_test drop column b; |
# Function & Procedure
# User Defined Procedure
1 | create procedure GetEmpInfo |
# User Defined Function
1 | create function GetHighSalEmp(@sal decimal(7, 2)) |
# isnull
isnull
fucntion is similar to Oracle nvl
function.
1 | select isnull(null, 'S'); --S |
# charindex
1 | select charindex('home', 'welcome home'); --9 |
# cast
1 | select 'abc' + cast(123 as varchar(10)); --abc123 |
# getdate
getdate
function is similar to Oracle sysdate
function.
1 | select getdate(); |
# dateadd
1 | select dateadd(day, 2, '2024-06-04'); --2024-06-06 00:00:00.000 |
# datediff
1 | select datediff(day, getdate(), dateadd(day, 2, getdate())); --2 |
# datepart
1 | select datepart(year, getdate()), datepart(week, getdate()); --2024 23 |
# T-SQL
Example Table
1 | -- Create table similar to scott.emp in SQL Server |
empno | ename | job | mgr | hiredate | sal | comm | deptno |
---|---|---|---|---|---|---|---|
7369 | SMITH | CLERK | 7902 | 1980-12-17 | 800.00 | NULL | 20 |
7499 | ALLEN | SALESMAN | 7698 | 1981-02-20 | 1600.00 | 300.00 | 30 |
7521 | WARD | SALESMAN | 7698 | 1981-02-22 | 1250.00 | 500.00 | 30 |
7566 | JONES | MANAGER | 7839 | 1981-04-02 | 2975.00 | NULL | 20 |
7698 | BLAKE | MANAGER | 7839 | 1981-05-01 | 2850.00 | NULL | 30 |
7782 | CLARK | MANAGER | 7839 | 1981-06-09 | 2450.00 | NULL | 10 |
7788 | SCOTT | ANALYST | 7566 | 1982-12-09 | 3000.00 | NULL | 20 |
7839 | KING | PRESIDENT | NULL | 1981-11-17 | 5000.00 | NULL | 10 |
7844 | TURNER | SALESMAN | 7698 | 1981-09-08 | 1500.00 | 0.00 | 30 |
7876 | ADAMS | CLERK | 7788 | 1983-01-12 | 1100.00 | NULL | 20 |
7900 | JAMES | CLERK | 7698 | 1981-12-03 | 950.00 | NULL | 30 |
7934 | MILLER | CLERK | 7782 | 1982-01-23 | 1300.00 | NULL | 10 |
# Variables
# Set Assignment
1 | declare @num int |
# Select Assignment
1 | declare @num int |
1 | declare @avg_sal decimal(7, 2) |
empno | ename | job | mgr | hiredate | sal | comm | deptno |
---|---|---|---|---|---|---|---|
7566 | JONES | MANAGER | 7839 | 1981-04-02 | 2975.00 | NULL | 20 |
7698 | BLAKE | MANAGER | 7839 | 1981-05-01 | 2850.00 | NULL | 30 |
7782 | CLARK | MANAGER | 7839 | 1981-06-09 | 2450.00 | NULL | 10 |
7788 | SCOTT | ANALYST | 7566 | 1982-12-09 | 3000.00 | NULL | 20 |
7839 | KING | PRESIDENT | NULL | 1981-11-17 | 5000.00 | NULL | 10 |
# Update Assignment
1 | declare @name nvarchar(50) |
# Table Variables
1 | declare @emp table ( |
# Condition Statement
# IF ELSE
1 | declare @price decimal(18,2) |
# WHEN THEN
1 | declare @today int |
# EXISITS
1 | declare @empno int = 7839 |
# While Loop
# Calculate the sum from 1 to 100.
1 | declare @a int |
# Mock Test Data
1 | declare @RowCount int = 0 |
# Cursor
1 | declare @empno int |
# Trigger
1 | create table #log |
# Custom Procedure
# With Output Parameter
1 | create procedure CalculateSum |
# Return
1 | create procedure CalculateSum |
# Custom Function
# Scalar Function
1 | create function CalculateSum(@a int, @b int) returns int |
# Table-valued Function
1 | create function GetHighSalEmp(@sal decimal(7, 2)) |
1 | create function GetHighSalEmp(@sal decimal(7, 2)) |
# SET NOCOUNT
Controls whether a message that shows the number of rows affected by a Transact-SQL statement or stored procedure is returned after the result set. This message is an additional result set.
1 | set nocount off |
# Misc.
# For XML Path
1 | select empno, ename, sal |
1 | <emp> |
1 | select ',' + ename |
1 | ,SMITH,ALLEN,WARD,JONES,BLAKE,CLARK,SCOTT,KING,TURNER,ADAMS,JAMES,MILLER |
# For XML Path + STUFF Implements STRING_AGG
STRING_AGG
is provided in SQL Server 2017 (14.x) and later
1 | select string_agg(ename, ',') |
STUFF(character_expression, start, length, replace_with_expression)
1 | select stuff( |
1 | SMITH, ALLEN, WARD, JONES, BLAKE, CLARK, SCOTT, KING, TURNER, ADAMS, JAMES, MILLER |
1 | select distinct |
job | enames | |
---|---|---|
ANALYST | SCOTT | |
CLERK | SMITH, ADAMS, JAMES, MILLER | |
MANAGER | JONES, BLAKE, CLARK | |
PRESIDENT | KING | |
SALESMAN | ALLEN, WARD, TURNER |
# Dynamic SQL
1 | exec('select * from emp') |
# Custom Types
1 | CREATE TYPE dbo.String FROM VARCHAR(255); |
name | system_type_id | user_type_id | schema_id | principal_id | max_length | precision | scale | collation_name | is_nullable | is_user_defined | is_assembly_type | default_object_id | rule_object_id | is_table_type |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
image | 34 | 34 | 4 | 16 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | ||
text | 35 | 35 | 4 | 16 | 0 | 0 | SQL_Latin1_General_CP1_CI_AS | 1 | 0 | 0 | 0 | 0 | 0 | |
uniqueidentifier | 36 | 36 | 4 | 16 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | ||
date | 40 | 40 | 4 | 3 | 10 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | ||
time | 41 | 41 | 4 | 5 | 16 | 7 | 1 | 0 | 0 | 0 | 0 | 0 | ||
datetime2 | 42 | 42 | 4 | 8 | 27 | 7 | 1 | 0 | 0 | 0 | 0 | 0 | ||
datetimeoffset | 43 | 43 | 4 | 10 | 34 | 7 | 1 | 0 | 0 | 0 | 0 | 0 | ||
tinyint | 48 | 48 | 4 | 1 | 3 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | ||
smallint | 52 | 52 | 4 | 2 | 5 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | ||
int | 56 | 56 | 4 | 4 | 10 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | ||
smalldatetime | 58 | 58 | 4 | 4 | 16 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | ||
real | 59 | 59 | 4 | 4 | 24 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | ||
money | 60 | 60 | 4 | 8 | 19 | 4 | 1 | 0 | 0 | 0 | 0 | 0 | ||
datetime | 61 | 61 | 4 | 8 | 23 | 3 | 1 | 0 | 0 | 0 | 0 | 0 | ||
float | 62 | 62 | 4 | 8 | 53 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | ||
sql_variant | 98 | 98 | 4 | 8016 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | ||
ntext | 99 | 99 | 4 | 16 | 0 | 0 | SQL_Latin1_General_CP1_CI_AS | 1 | 0 | 0 | 0 | 0 | 0 | |
bit | 104 | 104 | 4 | 1 | 1 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | ||
decimal | 106 | 106 | 4 | 17 | 38 | 38 | 1 | 0 | 0 | 0 | 0 | 0 | ||
numeric | 108 | 108 | 4 | 17 | 38 | 38 | 1 | 0 | 0 | 0 | 0 | 0 | ||
smallmoney | 122 | 122 | 4 | 4 | 10 | 4 | 1 | 0 | 0 | 0 | 0 | 0 | ||
bigint | 127 | 127 | 4 | 8 | 19 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | ||
hierarchyid | 240 | 128 | 4 | 892 | 0 | 0 | 1 | 0 | 1 | 0 | 0 | 0 | ||
geometry | 240 | 129 | 4 | -1 | 0 | 0 | 1 | 0 | 1 | 0 | 0 | 0 | ||
geography | 240 | 130 | 4 | -1 | 0 | 0 | 1 | 0 | 1 | 0 | 0 | 0 | ||
varbinary | 165 | 165 | 4 | 8000 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | ||
varchar |
167 |
167 | 4 | 8000 | 0 | 0 | SQL_Latin1_General_CP1_CI_AS | 1 | 0 | 0 | 0 | 0 | 0 | |
binary | 173 | 173 | 4 | 8000 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | ||
char | 175 | 175 | 4 | 8000 | 0 | 0 | SQL_Latin1_General_CP1_CI_AS | 1 | 0 | 0 | 0 | 0 | 0 | |
timestamp | 189 | 189 | 4 | 8 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | ||
nvarchar | 231 | 231 | 4 | 8000 | 0 | 0 | SQL_Latin1_General_CP1_CI_AS | 1 | 0 | 0 | 0 | 0 | 0 | |
nchar | 239 | 239 | 4 | 8000 | 0 | 0 | SQL_Latin1_General_CP1_CI_AS | 1 | 0 | 0 | 0 | 0 | 0 | |
xml | 241 | 241 | 4 | -1 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | ||
sysname | 231 | 256 | 4 | 256 | 0 | 0 | SQL_Latin1_General_CP1_CI_AS | 0 | 0 | 0 | 0 | 0 | 0 | |
String |
167 |
257 | 1 | 255 | 0 | 0 | SQL_Latin1_General_CP1_CI_AS | 1 | 1 | 0 | 0 | 0 | 0 |
1 | SELECT SelectedType.[name] AS SelectedTypeName |
SelectedTypeName | BaseTypeName | SelectedTypeMaxBytes | SelectedTypePrecision | SelectedTypeScale | SelectedTypeId | BaseTypeId |
---|---|---|---|---|---|---|
String | varchar | 255 | 0 | 0 | 0 | 0 |
# Include Execution Plan
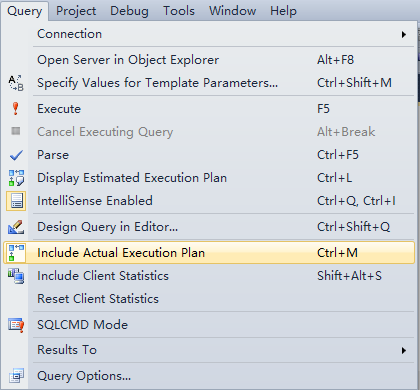